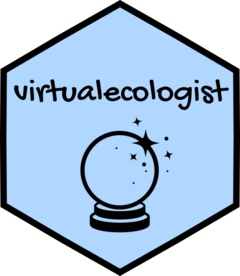
Identify potential next positions
potential_position_func.Rd
The function generates a bunch of potential positions to choose the next step from. Positions are generated based on provided bearing (Von Mises distribution) and step distributions (Gamma distribution). For each position, the value of resource_layer
is retrieved as well as the distance from the colony location.
Arguments
- n
Numeric, the number of potential positions to generate
- bearing
Numeric vector, mu and kappa to be passed on randomdir
- step
Numeric, shape and rate to be passed on randomdist
- from
data.frame including Lon and Lat fields and stepID of the point of origin
- colony_location
data.frame vector with lon and lat position of the colony
- resource_layer
SpatRaster, the suitability or any resource layer to be used to sort potential position (a single layer)
Value
A data.frame with the n potential positions from the point of origin, informed with the value of the provided environmental layer corresponding to each as well as the distance from the colony.
See also
Other individual movement functions:
randomdir()
,
randomdist()
,
simulate_trajectory_CPF()
,
simulate_trajectory_FR()
Examples
from <- data.frame(Lon = 1, Lat = 2, toto = 5)
colony_location <- data.frame(Lon = 2, Lat = 5)
cdt <- generate_env_layer(grid = create_grid(), n = 1)$rasters
#> [using unconditional Gaussian simulation]
potential_position_func(n = 10, from = from,
colony_location = colony_location, resource_layer = cdt,
bearing = c(90,10), step = c(4.5, 3))
#> angle step Lon Lat env dist_col
#> 1 124.54851 1.3176841 1.5797831 0.8167237 0.5954073 4.204329
#> 2 38.59724 1.5516632 1.9668066 3.2136489 0.5617037 1.786659
#> 3 317.19460 1.2513320 -0.2442748 2.1327102 0.6177145 3.641170
#> 4 185.32546 0.7604406 0.2398684 2.0216779 0.6177145 3.459547
#> 5 57.06428 0.5626446 1.4894966 2.2774203 0.5421721 2.770028
#> 6 208.31050 0.9354868 1.5323879 2.7692196 0.5329122 2.279264
#> 7 190.77661 3.2482543 -1.1179400 4.4628207 NA 3.163876
#> 8 180.25368 1.2824369 0.5149331 0.8128377 0.6540463 4.442719
#> 9 22.63236 0.2880789 0.7691424 1.8276799 0.5297902 3.402738
#> 10 111.86924 0.8619650 1.2896450 1.1881568 0.5954073 3.877467
#> potential_position_id
#> 1 1
#> 2 2
#> 3 3
#> 4 4
#> 5 5
#> 6 6
#> 7 7
#> 8 8
#> 9 9
#> 10 10